Container in Flutter – কন্টেইনার ক্লাস এক বা একাধিক উইজেট সংরক্ষণ করতে এবং আমাদের সুবিধা অনুযায়ী স্ক্রিনে এটি স্থাপন করতে ব্যবহার করা হয়।
Container একটি বাক্সের মতো মূলত উইজেট সংরক্ষণ করার জন্য ব্যবহার করা হয় । যা একটি উইজেট সংরক্ষণ করে তার একটি মার্জিন থাকে, যা বর্তমান উইজেট কে অন্যান্য বিষয়বস্তুর সাথে আলাদা করে।
Properties of Container Class:
Container({Key key,
AlignmentGeometry alignment,
EdgeInsetsGeometry padding,
Color color,
Decoration decoration,
Decoration foregroundDecoration,
double width,
double height,
BoxConstraints constraints,
EdgeInsetsGeometry margin,
Matrix4 transform,
Widget child,
Clip clipBehavior: Clip.none});
1. child: কন্টেইনার উইজেটের একটি ইলিমেন্ট ‘child‘ যা তার child দের সংরক্ষণ করে। child ক্লাস যেকোনো উইজেট হতে পারে। Container এতে একটি টেক্সট উইজেট নেওয়ার উদাহরণ দেওয়া যাক।
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text(
"AppBar Heading",
style: TextStyle(color: Colors.amber),
),
),
body: Container(height: 110, width: 110, margin: const EdgeInsets.all(10), color: Colors.amber,
child: const Text(
"i am Text in a Container",
maxLines: 2,
),
),
),
);
}
}
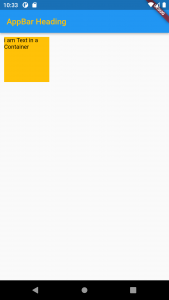
2. Color: রঙের বৈশিষ্ট্য সমগ্র ধারকটির পটভূমির রঙ সেট করে। এখন আমরা একটি পটভূমির রঙ ব্যবহার করে ধারকটির অবস্থান কল্পনা করতে পারি।
style: TextStyle(color: Colors.amber, backgroundColor: Colors.black),
3. Container height and width
Container(height: 110, width: 220, margin: const EdgeInsets.all(10), color: Colors.amber)
width: double.infinity
4. Container margin, padding
body: Container(
height: 200,
width: double.infinity,
color: Colors.purple,
margin: EdgeInsets.all(20),
padding: EdgeInsets.all(30),
child: Text("Hello! i am inside a container!",
style: TextStyle(fontSize: 20)),
),
margin: EdgeInsets.all(20), হল left, top, right, bottom সকল দিকে ২০ মারজিন। কাস্টাম করে দিতে চাইলে margin: EdgeInsets.only(left, top, right, bottom) এমন স্টাকচারে দিতে হয়।
5. Alignment : আমরা বিভিন্ন উপায়ে Alignment করতে পারি: নীচে, নীচে কেন্দ্র, বাম, ডান, ইত্যাদি এখানে শিশুটি নীচের কেন্দ্রে Alignment করা হয়েছে।
body: Container(
height: 200,
width: double.infinity,
color: Colors.purple,
alignment: Alignment.bottomCenter,
margin: EdgeInsets.all(20),
padding: EdgeInsets.all(30),
child: Text("Hello! i am inside a container!",
style: TextStyle(fontSize: 20)),
),
alignment: Alignment.bottomCenter,
6. Decoration: BoxDecoration বাক্স সাজাইতে ব্যবহার করা হয় । এই পেইন্ট শিশুর পিছনে. যেখানে ফোরগ্রাউন্ড ডেকোরেশন একটি child সামনে রং করে।
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("Container example"),
),
body: Container(
height: 200,
width: double.infinity,
//color: Colors.purple,
alignment: Alignment.center,
margin: EdgeInsets.all(20),
padding: EdgeInsets.all(30),
decoration: BoxDecoration(
border: Border.all(color: Colors.black, width: 3),
),
child: Text("Hello! i am inside a container!",
style: TextStyle(fontSize: 20)),
),
),
);
}
}
border: Border.all(color: Colors.black, width: 3),
8. transform: কন্টেইনারের এই বৈশিষ্ট্য আমাদের কন্টেইনার ঘোরাতে সাহায্য করে। আমরা যে কোনো অক্ষে ধারকটিকে ঘোরাতে পারি, এখানে আমরা z-অক্ষে ঘোরে।
transform: Matrix4.rotationZ(0.1)
9. constraints: When we want to give additional constraints to the child, we can use this property.
10. clipBehaviour : This property takes in Clip enum as the object. This decides whether the content inside the container will be clipped or not.
11. foregroundDecoration: This parameter holds Decoration class as the object. It controls the decoration in front of the Container widget.
- Container in Flutter